OpenWISP Dashboard
Note
This documentation page is aimed at developers who want to customize, change or extend the code of OpenWISP Utils in order to modify its behavior (e.g.: for personal or commercial purposes or to fix a bug, implement a new feature or contribute to the project in general).
If you aren't a developer and you are looking for information on how to use OpenWISP, please refer to:
The admin_theme
sub app of this package provides an admin dashboard
for OpenWISP which can be manipulated with the functions described in the
next sections.
Example taken from the Controller Module:
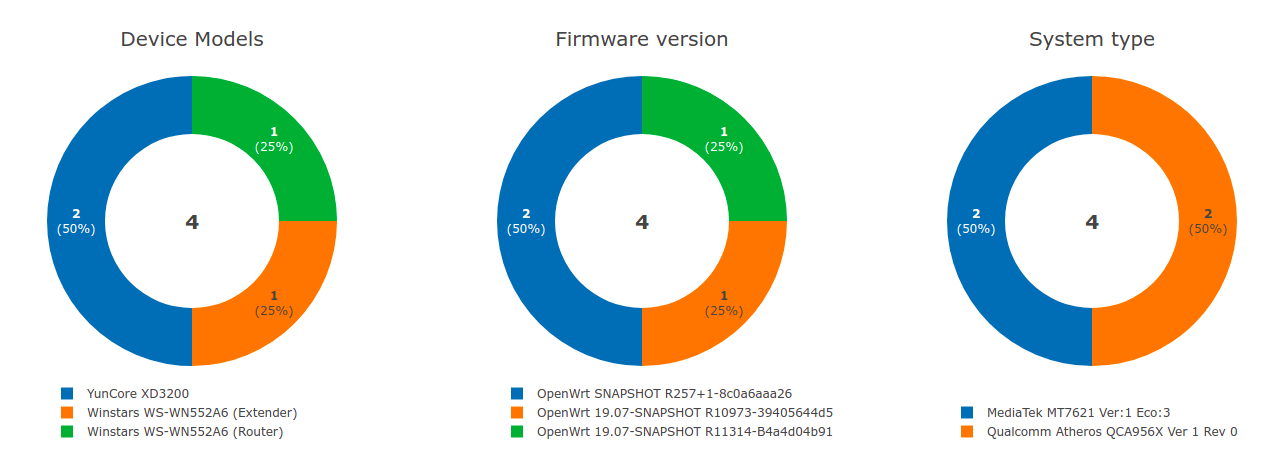
register_dashboard_template
Allows including a specific django template in the OpenWISP dashboard.
It is designed to allow the inclusion of the geographic map shipped by OpenWISP Monitoring but can be used to include any custom element in the dashboard.
Note
It is possible to register templates to be loaded before or after
charts using the after_charts
keyword argument (see below).
Syntax:
register_dashboard_template(position, config)
Parameter |
Description |
|
( |
|
( |
|
optional ( |
|
optional ( |
Following properties can be configured for each template config
:
Property |
Description |
|
( |
|
( |
|
( |
Code example:
from openwisp_utils.admin_theme import register_dashboard_template
register_dashboard_template(
position=0,
config={
"template": "admin/dashboard/device_map.html",
"css": (
"monitoring/css/device-map.css",
"leaflet/leaflet.css",
"monitoring/css/leaflet.fullscreen.css",
),
"js": (
"monitoring/js/device-map.js",
"leaflet/leaflet.js",
"leaflet/leaflet.extras.js",
"monitoring/js/leaflet.fullscreen.min.js",
),
},
extra_config={
"optional_variable": "any_valid_value",
},
after_charts=True,
)
It is recommended to register dashboard templates from the ready
method of the AppConfig of the app where the templates are defined.
unregister_dashboard_template
This function can be used to remove a template from the dashboard.
Syntax:
unregister_dashboard_template(template_name)
Parameter |
Description |
|
( |
Code example:
from openwisp_utils.admin_theme import unregister_dashboard_template
unregister_dashboard_template("admin/dashboard/device_map.html")
An ImproperlyConfigured
exception is raised the specified dashboard
template is not registered.
register_dashboard_chart
Adds a chart to the OpenWISP dashboard.
At the moment only pie charts are supported.
The code works by defining the type of query which will be executed, and optionally, how the returned values have to be colored and labeled.
Syntax:
register_dashboard_chart(position, config)
Parameter |
Description |
|
( |
|
( |
Following properties can be configured for each chart config
:
Property |
Description |
|
It is a required property in form of |
|
An optional |
|
An optional |
|
An optional |
|
An optional |
|
An optional |
|
An optional Note: The chart legend is disabled if configuration for quick link button is provided. |
Dashboard Chart query_params
Property |
Description |
|
( |
|
( |
|
( |
|
( |
|
Alternative to |
|
Alternative to |
|
|
|
( |
Dashboard chart quick_link
Property |
Description |
|
( |
|
( |
|
( |
|
( |
Code example:
from openwisp_utils.admin_theme import register_dashboard_chart
register_dashboard_chart(
position=1,
config={
"query_params": {
"name": "Operator Project Distribution",
"app_label": "test_project",
"model": "operator",
"group_by": "project__name",
},
"colors": {"Utils": "red", "User": "orange"},
"quick_link": {
"url": "/admin/test_project/operator",
"label": "Open Operators list",
"title": "View complete list of operators",
"custom_css_classes": ["negative-top-20"],
},
},
)
For real world examples, look at the code of OpenWISP Controller and OpenWISP Monitoring.
An ImproperlyConfigured
exception is raised if a dashboard element is
already registered at same position.
It is recommended to register dashboard charts from the ready
method
of the AppConfig of the app where the models are defined. Checkout app.py
of the test_project
for reference.
unregister_dashboard_chart
This function can used to remove a chart from the dashboard.
Syntax:
unregister_dashboard_chart(chart_name)
Parameter |
Description |
|
( |
Code example:
from openwisp_utils.admin_theme import unregister_dashboard_chart
unregister_dashboard_chart("Operator Project Distribution")
An ImproperlyConfigured
exception is raised the specified dashboard
chart is not registered.