Sending Commands to Devices
Default command options
By default, there are three options in the Send Command dropdown:
Reboot
Change Password
Custom Command
While the first two options are self-explanatory, the custom command option allows you to execute any command on the device as shown in the example below.
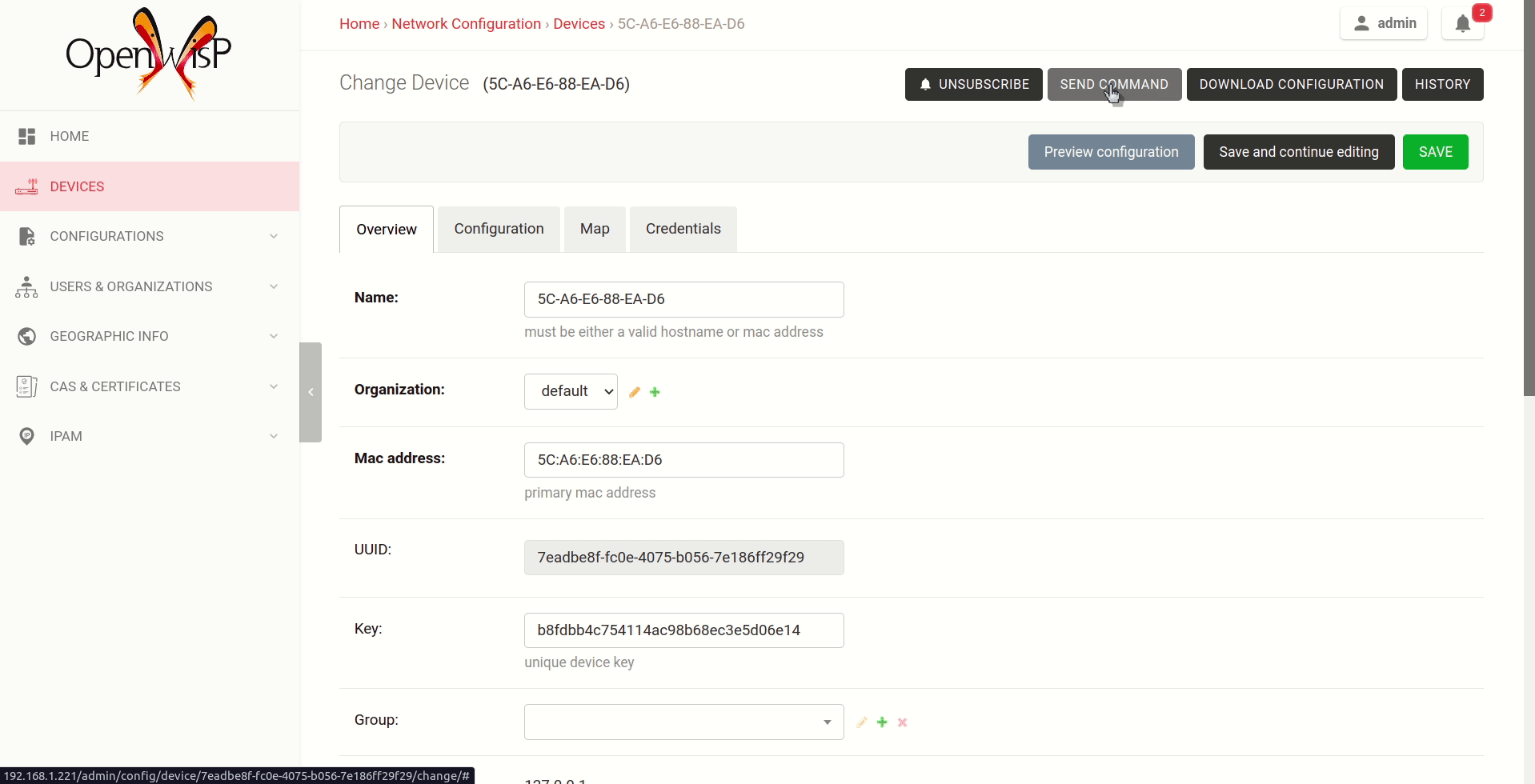
Note: in order for this feature to work, a device needs to have at least one Access Credential (see How to Configure Push Updates).
The Send Command button will be hidden until the device has at least one Access Credential.
If you need to allow your users to quickly send specific commands that are used often in your network regardless of your users’ knowledge of Linux shell commands, you can add new commands by following instructions in “How to define new options in the commands menu” section below.
If you are an advanced user and want to register commands programatically, then refer to “Register / Unregistering commands” section.